Python programming
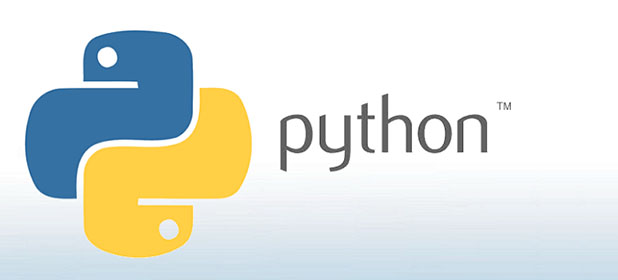
4 Techniques for Testing Python Command-Line (CLI) Apps
Moving to Python 3 (from Python 2)
An Introduction to pyttsx3: A Text-To-Speech Converter for Python
10 Interesting Python Tricks to knock your socks off
Python Scope & the LEGB Rule: Resolving Names in Your Code
Processing XML in Python — ElementTree
stevedore – Manage Dynamic Plugins for Python Applications
Python Bindings: Calling C or C++ From Python
Object-oriented programming in Python
Three Decorators Commonly Used in Python Custom Classes
Matplotlib and Plotly
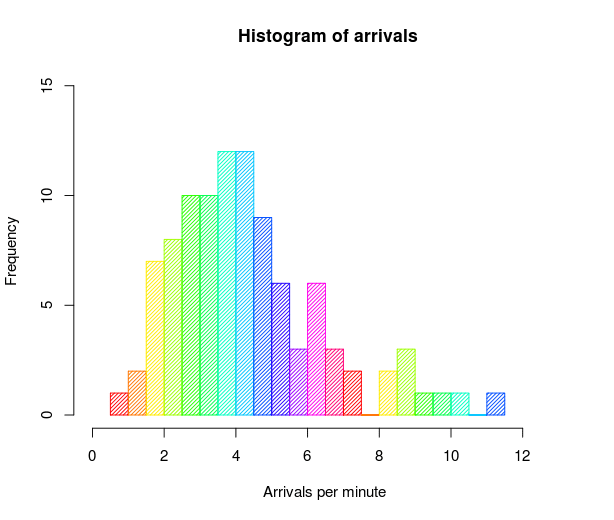
Histograms in Matplotlib
Learn about histograms and how you can use them to gain insights from data with the help of matplotlib.
Plot histogram with specific color, edge color and line width
Forget Matplotlib! You Should Be Using Plotly
GUIs with Python
Building A Real-time Dashboard Using Python Plotly Library And Web Service
Python Progress Bars with Tqdm by Example
Python programming with the Visual Studio Code IDE
Visual Studio Code is a lightweight but powerful source code editor which runs on your desktop and is available for Windows, macOS and Linux. It comes with built-in support for JavaScript, TypeScript and Node.js and has a rich ecosystem of extensions for other languages (such as C++, C#, Java, Python, PHP, Go) and runtimes (such as .NET and Unity).
Getting Started with Python in VS Code
Tutorial
- Learn Python Programming: The Definitive Guide
- Python 2.7 Tutorial
- Python Challenge is a game in which each level can be solved by a bit of (Python) programming
- Shichao’s PER Notes based on the book Python Essential Reference
Approfondimenti su Python
- Python3 Built-in Types
- Performing String and Bytes Data Conversion in Python3.x
- Byte Objects vs String in Python
- Python Bytes, Bytearray
- Python map() function
- Python zip()
- Python lambda
- What you should know about the Python list
- struct — Interpret strings as packed binary data
This module performs conversions between Python values and C structs represented as Python strings. This can be used in handling binary data stored in files or from network connections, among other sources. It uses Format Strings as compact descriptions of the layout of the C structs and the intended conversion to/from Python values. - Python Exceptions: An Introduction
Discussioni sul linguaggio
- Guido van Rossum Quits As Python BDFL
- Python Passion For Assignment Expressions – PEP 572
- PEP 484 — Type Hints
Jupyter Notebooks
- How to Start and Run a Jupyter Notebook
- Jupyter Notebook for Beginners: A Tutorial
- A gallery of interesting Jupyter Notebooks
- Why Python and Jupyter Notebooks?
- How to Setup Your JupyterLab Project Environment
- Jupyter Notebooks on Various Topics
- Project Jupyter: Architecture and Evolution of an Open Platform for Modern Data Science (slides)
- Getting Started with PySpark for Big Data Analytics, using Jupyter Notebooks and Docker
- 10 Simple hacks to speed up your Data Analysis in Python
- An argument against using Jupyter Notebook for Machine Learning
Examples of IPython Notebooks for science:
- LIGO Gravitational Wave Data
- Satellite Imagery Analysis
- 12 Steps to Navier-Stokes
- Computer Vision
- Machine Learning
Librerie e tool
- Una review delle librerie Python più popolari
- numpy, a Python package for scientific computing
- matplotlib, 2D plotting library which produces publication quality figures
- cartopy, library providing cartographic tools for Python
- netcdf4-python, a Python interface to the netCDF-C library
- JSON encoder and decoder
- A simple GUI designer for the python tkinter module
- Make Your Own Hex Viewer (in Python)
- pyexcel – Let you focus on data, instead of file formats
Single API for reading, manipulating and writing data in csv, ods, xls, xlsx and xlsm files - A Python implementation of the Quine McCluskey algorithm
- How to use GitHub as a PyPi server
- argparse — Parser for command-line options, arguments and sub-commands
- Pweave – Scientific Reports Using Python
Pweave is a scientific report generator and a literate programming tool for Python. Pweave can capture the results and plots from data analysis and works well with NumPy, SciPy and matplotlib. It is able to run python code from source document and include the results and capture matplotlib plots in the output. - Basics of Sweave and Pweave
Q&A da stackoverflow
- What does if __name__ == “__main__”: do?
- How to return dictionary keys as a list in Python?
- Watch out for list(dict.keys()) in Python 3
- What does a leading `\x` mean in a Python string `\xaa`
- Python Comparison of byte literals
- What’s the correct way to convert bytes to a hex string in Python 3?
- Python3 bytes to hex string
- Byte to Hex and Hex to Byte String Conversion (Python recipe)
- How can I check if a string represents an int, without using try/except?
- Executing and killing a shell process using python script
- Python Copy Through Assignment?
- Break A List Into N-Sized Chunks
- Efficiently finding the longest matching prefix string
- Bar Plot In MatPlotLib
- Getting the same subplot size using matplotlib imshow and scatter
- Class vs. Type in Python
- Length of an integer in Python
- Python Bottle and Cache-Control
- Setting cookies for static files using bottle.py
- Python flask writing to a csv file and reading it
- How do I fix the “image ”pyimage10“ doesn’t exist” error, and why does it happen?
- How can I make a time delay in Python?
- File as command line argument for argparse – error message if argument is not valid
- How to send EOF to Python sys.stdin from commandline? CTRL-D doesn’t work
- Python timeit command-line error: “SyntaxError: EOL while scanning string literal”
- How to use timeit module
- Why is “1000000000000000 in range(1000000000000001)” so fast in Python 3?
- List of Modules (>>> help(‘modules’) not working)
- Pytesseract : “TesseractNotFound Error: tesseract is not installed or it’s not in your path”, how do I fix this?
Python Programming Tips
- How to run multiple Python versions on Windows
- How we suggest command lining
- How to run external programs from Python and capture their output
- Calling an external command in Python
- Getting realtime output using Python Subprocess
- 3 Ways to Write Text to a File in Python
- Processing Text Files in Python 3
- How To Handle Plain Text Files in Python 3
- [Tkinter] tkinter calendar widget
- Quick Tip: The easiest way to grab data out of a web page in Python
- Converting Int/Float ↔ byte stream in python
- Python Concepts/Console Input
- You can use the argparse package to easily turn a python script into a command-line program
- The Why, When, and How of Using Python Multi-threading and Multi-Processing
- Python Recursion by Example
- How to Use Lambda for Efficient Python Code
- Make Your Code Great, Python Style
- When to Not Use Lists in Python
- 8 Python Dictionary Things I Regret Not Knowing Earlier
Python and Cloud Computing
Python Frameworks for web applications
- Python3 native http.server
- Python http.server and upstart-socket-bridge
- Web Frameworks for Python
- Python-driven Web Applications
- Simple Python HTTP(S) Server — Example
- Using Python to Setup a Simple Server
- How do I forward a request to a different url in python
- Creating a Python3 Webserver From the Ground Up
- Using Streamlit to create interactive WebApps from simple Python scripts
Flask
helloflask/flask-examples on GitHub
Bottle
Bottle is a WSGI micro web-framework for the Python programming language. It is designed to be fast, simple and lightweight, and is distributed as a single file module with no dependencies other than the Python Standard Library.
- Bottle web framework Tutorial
- Bottle API Reference
- Python bottle.response.set_header() Examples
- Bottle Recipes
- Top 10 Python Frameworks for Web Development in 2019
- Putting Bottle in a Container: Docker and Bottle
- Session Management in Bottle
- Beaker
Beaker is a web session and general caching library that includes WSGI middleware for use in web applications. - Bottle.py session with Beaker
- Bottle – Primer to Asynchronous Applications
- Python — Bottle : An Asynchronous Application
- Proposal for asynchronous responses in Bottle (2010)
- Chattle Chat App
Chattle is a small chat app to demonstrate the use of asynchronous responses with the webframework Bottle. It uses gevent to allow many concurrent connections from chat clients to the chat server. - Build beautiful, web-based analytics applications with Dash
Other WSGI-compatible web frameworks (CherryPi, mod_wsgi, uWSGI)
- Web Server Gateway Interface
- An Introduction to the Python Web Server Gateway Interface (WSGI)
- WSGI Servers
- Using Python, Flask, and Angular to Build Modern Web Apps – Part 1
Network Programming with Python
- Socket Programming HOWTO
- Network Programming & Automation
- Multicast with Python
- User Datagram Client and Server in Python
- Sending & Sniffing WLAN Beacon Frames using Scapy
Python and Data Science
- Why Should We Use NumPy?
- Getting Started with PySpark for Big Data Analytics, using Jupyter Notebooks and Docker
- We need more Interactive Data Visualization tools (for the Web) in Python
- The Next Level of Data Visualization in Python
- Interpreting Data through Visualization with Python Matplotlib
- I was looking for a house, so I built a web scraper in Python! – Part I
- I was looking for a house, so I built a web scraper in Python! — part II (EDA)
- Locally-Linear Embedding in Python
- Minimal Pandas Subset for Data Scientists
- Forget APIs Do Python Scraping Using Beautiful Soup, Import Data File from the web: Part 2
- Understanding ROC Curves with Python
Python e Matematica
- Symbolic Computing Using Python: Part 1-Basics with SymPy
- Tutorial: A Simple Framework For Optimization Programming In Python Using PuLP, Gurobi, and CPLEX
Image Processing in Python
- cImage – A simple image processing library for Python educators.
- PIL/Pillow – PIL (Python Imaging Library) adds many image processing features to Python. Pillow is a fork of PIL that adds some user-friendly features.
Sound Processing in Python
- CSC111 JES Page on Sound Processing
- Audio and Digital Signal Processing(DSP) in Python
- [Elegant SciPy book] Chapter 4. Frequency and the Fast Fourier Transform
- Data Augmentation Techniques for Audio Data in Python
- CVxTz (2018). Audio data augmentation in Kaggle Notebooks
- Torchaudio is a library for audio and signal processing with PyTorch. It provides I/O, signal and data processing functions, datasets, model implementations and application components.
- Speech Recognition with Wav2Vec2 (and PyTorch)
This tutorial shows how to perform speech recognition using using pre-trained models from wav2vec 2.0 [paper].
Audio Libraries for Python
- Sound-playing interface for Windows in Python (winsound)
- pygame.midi
PyGame module for interacting with midi input and output. - PyAudio with PortAudio for Windows
- PyAudio
PyAudio provides Python bindings for PortAudio, the cross-platform audio I/O library. With PyAudio, you can easily use Python to play and record audio on a variety of platforms, such as GNU/Linux, Microsoft Windows, and Apple Mac OS X / macOS.
Multimedia applications in Python
- Using Python for Voice over IP
- Light weight implementations of real-time communication protocols and applications in Python
- Pygame
- Pygame Introduction
Python and IoT
Object-oriented programming in Python
- Behind the scenes: Relationship between Class and type
- A brief tour of Python 3.7 data classes
- Supercharge Your Classes With Python super()
- Python’s super() considered super!
- Understanding Python super() with __init__() methods
Log Analysis in Python
Programmazione avanzata in Python
- Introduzione alla programmazione parallela e concorrente con Python
- Simple Examples of Concurrent Server Design in Python
- http://www.cs.ubbcluj.ro/~dadi/compnet/labs/lab2/example-python-numbergguess-concurrent-threads.html
- How to make a simple multithreaded socket server in Python that remembers clients
- Let’s Build A Web Server. Part 3
- Python: How To Keep Your Passwords Safe?
- MD5 hash in Python
Tools sviluppati in Python
Performance
- How to use timeit module
- Timeit in Python with Examples
- On Random vs. Streaming I/O Performance; Or Seek(), and You Shall Find – Eventually
- Python: How To Reduce Memory Consumption By Half By Adding Just One Line Of Code?
- Memory Management in Python
Uso di Python in ambienti particolari
- Compiling & Installing Python 2.7 on the Netgear ReadyNAS Duo2
- Precompiled Python wheels for ARM
- FreeZTP: Zero-Touch Provisioning for Cisco Catalyst
- FreeZTP: An Open-Source Zero-Touch Provisioning System for Cisco IOS.